Gutenberg, the block editor for WordPress, has revolutionized the way we create and manage content on our websites. With its flexible block-based approach, it allows for endless customization possibilities. One of the key features that makes this flexibility possible is the use of JavaScript filter hooks. These hooks enable developers to modify and enhance the behavior of Gutenberg blocks, making it easier than ever to tailor the editor to your specific needs.
In this guide, we will explore how to leverage JavaScript filter hooks in Gutenberg, empowering you to take control of your content creation experience and create dynamic, personalized web content effortlessly. Whether you’re a seasoned WordPress developer or just getting started with Gutenberg, understanding how to use filter hooks in JavaScript is a valuable skill that will open up new horizons for your web development projects.
What are filter hooks?
Filter hooks in the context of WordPress and Gutenberg are a fundamental part of the platform’s plugin and theme development architecture. They provide a way to modify or filter the data or behavior of various components within WordPress and Gutenberg without directly altering their core code. These hooks serve as entry points where developers can intervene and apply custom code to change the behavior, appearance, or data of WordPress and Gutenberg elements.
Filter hooks typically follow a common pattern: a function or method is defined, and this function accepts one or more arguments, processes them, and then returns the modified value. Other parts of the code can “hook into” these filters, providing their custom code to influence the final output.
For instance, in Gutenberg, filter hooks can be used to alter the attributes, content, or appearance of individual blocks, modify editor settings, or even create entirely new custom blocks with unique functionality. By using filter hooks, developers can extend and customize the capabilities of WordPress and Gutenberg without the need to modify the core code, making their solutions more maintainable and compatible with future updates. This flexibility is what makes WordPress such a powerful and adaptable platform for web development and content management.
When a filter hook is needed
Filter hooks are needed in various situations where you want to modify, enhance, or customize the behavior, appearance, or data of WordPress or Gutenberg components without directly altering their core code. Here are some common scenarios where filter hooks are beneficial:
- Customization: If you want to change the default behavior or appearance of a particular WordPress or Gutenberg element, such as a block in the editor, the appearance of a post, or the output of a function, you can use filter hooks to apply your custom modifications.
- Plugin and Theme Development: When developing WordPress plugins or themes, filter hooks allow you to make your code extensible and compatible with other developers’ solutions. You can create filter hooks within your plugins or themes to enable other developers to customize your functionality.
- Third-Party Integration: When integrating third-party services or APIs into your WordPress site, filter hooks can be used to adjust the data or parameters sent to external services or to process the data received from them.
- Content Manipulation: Filter hooks can be used to modify content before it is displayed, such as adding or removing specific elements from posts, pages, or other content types.
- Data Validation and Sanitization: Filter hooks are essential for ensuring the security and data integrity of your WordPress site. You can use them to validate and sanitize user input or other data before it is saved in the database.
- Performance Optimization: Filter hooks can be employed to improve the performance of your website by caching or altering data retrieval and rendering processes.
- Localization: If you’re building a multilingual website, filter hooks can help in translating or customizing the text strings displayed on your site.
- Accessibility: You can use filter hooks to enhance the accessibility of your site by modifying HTML output or adding ARIA attributes to elements.
- SEO Customization: Filter hooks enable you to adjust meta tags, title tags, and other SEO-related content to improve your site’s search engine optimization.
In summary, filter hooks are needed whenever you want to customize or extend WordPress and Gutenberg functionality in a way that allows for flexibility, maintainability, and compatibility with other themes and plugins. They provide a structured and organized way to modify the behavior and appearance of your WordPress site to meet your specific requirements.
How a filter hook works
To understand the functionality of a filter hook, let’s make a real example. Suppose, we want to add a custom class to the existing default paragraph block. let’s figure out – how can we do it.
Since we are talking about the Javascript filter hooks, we need to create a javascript file where we can keep our filter hook codes. To do this, you can either use your theme or a custom plugin whatever you like. I hope you have a javascript file, for example, I called it “custom-hook.js”
To add a custom class to the paragraph block, you can use the blocks.getSaveContent.extraProps
filter. This filter allows you to add extra props to the element saved by the block.
Add the following codes to the file (custom-hook.js):
import { addFilter } from '@wordpress/hooks';
function addCustomClass( extraProps, blockType, attributes ) {
if ( blockType.name === 'core/paragraph' ) {
// Add the custom class
extraProps.className = ( extraProps.className || '' ) + ' my-custom-class';
}
return extraProps;
}
addFilter(
'blocks.getSaveContent.extraProps',
'my-plugin/add-custom-class',
addCustomClass
);
In this example, the addCustomClass
function is added as a filter for the blocks.getSaveContent.extraProps
hook. This function is called when the paragraph block is saved, and it adds the my-custom-class
class to the block.
Remember to replace 'my-plugin/add-custom-class'
with your actual plugin and filter name.
Alternative Hook
We can also do the same task using a different hook. We can use the editor.BlockEdit
filter for this purpose. Here’s an example of how to add a custom class to the paragraph block:
wp.hooks.addFilter('editor.BlockEdit', 'my-custom-attributes', (BlockEdit) => {
return (props) => {
if (props.name === 'core/paragraph') {
// Add a custom class to the block.
props.attributes.className = `${props.attributes.className} my-custom-class`;
}
return <BlockEdit {...props} />;
};
});
In this code:
- We use the
editor.BlockEdit
filter to modify the block editor. - We check if the block being edited is the “core/paragraph” block by inspecting
props.name
. - We add a custom class to the paragraph block’s
className
attribute. - We add a custom data attribute with a value to the paragraph block.
Now, make sure you have enqueued the file successfully. To enqueue the file, you can follow the below code structure:-
function enqueue_custom_paragraph_attributes() {
wp_enqueue_script('custom-paragraph-attributes', FILE_PATH, array('wp-blocks'));
}
add_action('enqueue_block_editor_assets', 'enqueue_custom_paragraph_attributes');
Note: FILE_PATH – replace it based on your theme or plugin
Finally, if you do the all steps, now checkout the default paragraph block and you will find a new class.
Optimization
I hope you have already noticed that our custom class is added but the same class has been added multiple times that is not our expected behavior. Because we want the custom class only 1 time. So, to fix the issue, you have to modify your previous codes. If you modify our latest filter hook codes, it will look like the following codes:-
wp.hooks.addFilter('editor.BlockEdit', 'my-custom-attributes', (BlockEdit) => {
let addedCustomClass = false;
return (props) => {
if (props.name === 'core/paragraph' && !addedCustomClass) {
// Check if className is defined, and then add the custom class.
props.attributes.className = (props.attributes.className || '') + ' my-custom-class';
// Set a flag to prevent adding the class again.
addedCustomClass = true;
}
return <BlockEdit {...props} />;
};
});
In this modified code:
- We introduce a variable
addedCustomClass
that is initially set tofalse
. - Within the filter hook, we check if the block being edited is a paragraph block (
props.name === 'core/paragraph'
) and ifaddedCustomClass
isfalse
. If both conditions are met, we add the custom class and setaddedCustomClass
totrue
to prevent it from being added again. - We use
(props.attributes.className || '')
to ensure that theclassName
attribute is defined. If it’sundefined
, an empty string''
is used to avoid adding anundefined
class.
This modification ensures that the custom class is added only once to the paragraph block, regardless of how many times you edit it.
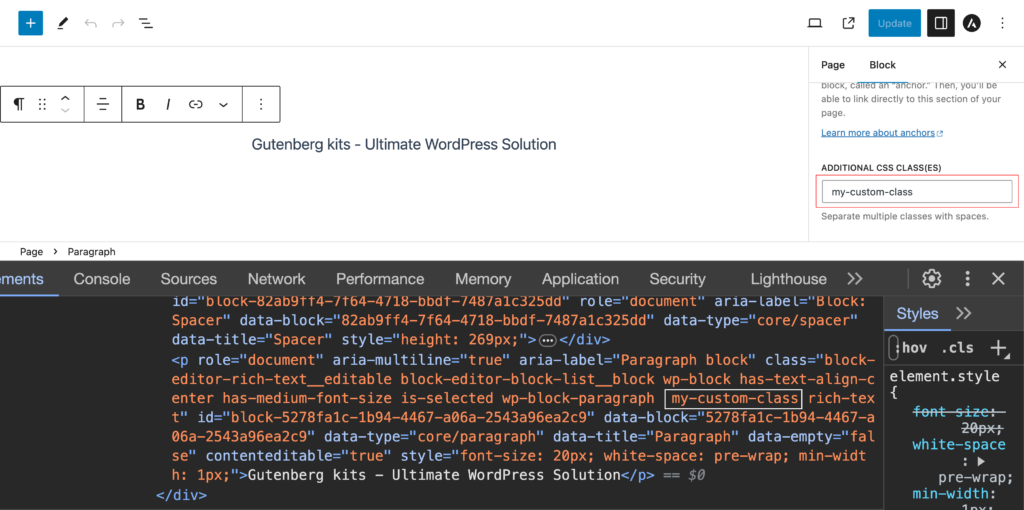
I Highly recommend visiting the Gutenberg official handbook on block filter hooks.
Conclusion
In conclusion, harnessing the power of JavaScript filter hooks in Gutenberg is a vital skill for WordPress developers seeking to extend and customize the behavior and appearance of the block editor. This guide has walked you through the essential steps to utilize filter hooks effectively, using the example of adding custom attributes to the default paragraph block.
By understanding how to create and apply filter hooks, you can tailor your WordPress site to meet your specific requirements without resorting to complex, core code modifications. This not only enhances the flexibility of your site but also ensures compatibility with future updates and other plugins or themes.
JavaScript filter hooks empower you to take control of your content creation experience, opening up a world of possibilities for creating dynamic, personalized web content effortlessly. As you continue to explore the capabilities of WordPress and Gutenberg, filter hooks will remain a valuable tool in your developer’s toolkit, enabling you to build websites that are both highly functional and uniquely tailored to your needs.